- Published on
TypeScript - 基本类型注释
- Authors
- Name
- Deng Hua
目录
可变类型
为变量分配基本类型很容易。只需在变量名后添加 Type
即可
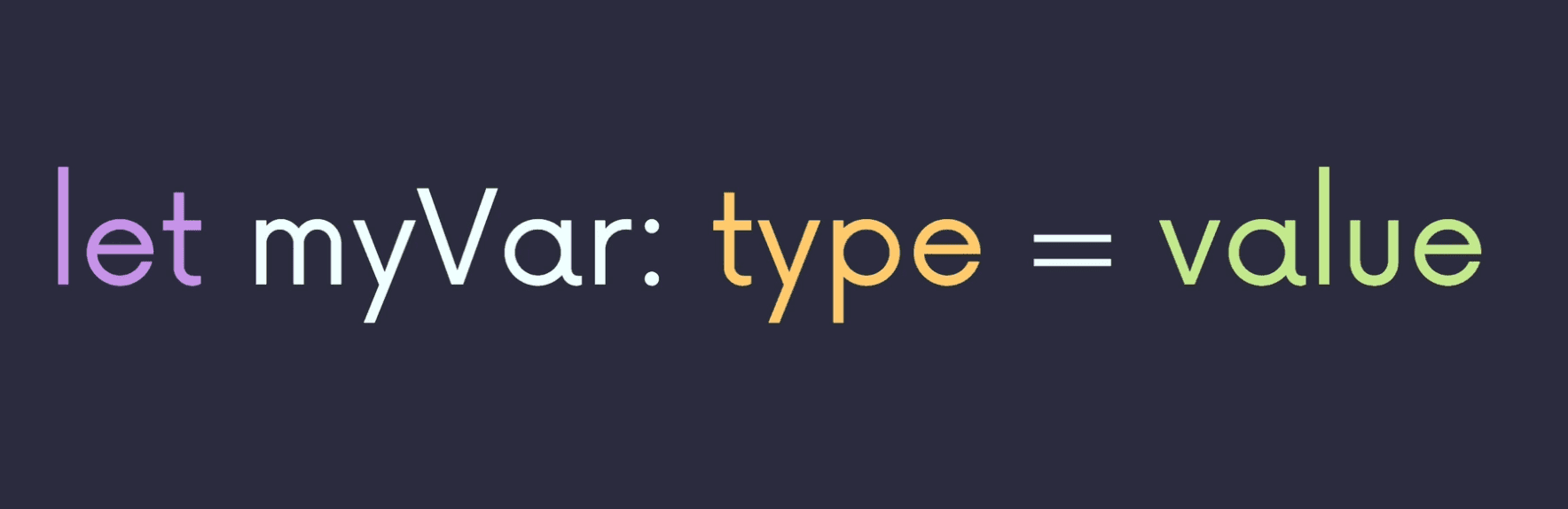
// Declaring a variable in JS
const awesomeVariable = 'So Awesome!';
// Declaring a variable in TS
const myAwesomeVariable: string = 'So Awesome';
再上述示例中,我们为myAwesomeVariable
常量赋与了一个String
类型。
由于TS已经知道变量的类型,后续对此变量赋值不是该类型的值,抑或是调用了不属于此类型的方法,便会作出对应报错或是提示。
let movieTitle: string = 'Amadeus';
movieTitle = 'Arrival';
movieTitle = 9; // error: 不能将类型“number”分配给类型“string”。
movieTitle.uppercase // error: 属性“uppercase”在类型“string”上不存在。你是否指的是“toUpperCase”?```
Number类型 与 Boolean类型
当然,在Typescript中,不只有一个类型。
Number
一些编程语言有许多数字类型-如float
, int
, etc
。在Typescript(以及Javascript)中,数字类型只有一个,即numbers
。 数字可以用简单的数字类型注释。
let myNumber: number = 42;
myNumber = "I'm a string"; // ✅ Can't reassign to a difference type
myNumber = 60; // ❌ Can reassign to a value of same type
Booleans
布尔值表示真值true
或假值false
,且仅有这个值。 布尔值可以用简单的布尔类型注释。
const myBoolean : boolean = true;
myBoolean = 87;// ✅ Can't reassign to a difference type
myBoolean = false; // ❌ Can reassign to a value of same type
类型推断
Type Inference refers to the Typescript compiler's ability to infer types from certain values in your code.
Typescript can remember a value's type even if you didn't provide a type annotation, and it will enforce that type moving forward.
大多数情况下,即使没有显式注释类型,TS也可以自动进行类型推断。
let x = 27; // 创建了一个变量,但没有进行类型注释。
x = 'Twenty-seven'; // ❌ 不能将类型“string”分配给类型“number”
第一次赋值时,TS会推断x
的类型是一个number
类型。当更改为一个类型为string
的值时,就会报错。
这也对我们日常的编码有一定的便利,一定意义上可以省略一些类型注释。
另一个例子
let isFunny = false;
isFunny = true; // <- type inference
isFunny = 'asd'; // ❌ 不能将类型“string”分配给类型“boolean”
Any 类型
'any' is an escape hatch! It turns off type checking for this variable so you can do your thing. NOTE: it sort of defeats the purpose of TS and types, so use it sparingly
Any
是Typescript里的一种特殊类型,使用它就意味着,你不希望TS进行任何类型检查。
let thing: any = "Hello";
thing = 1; // ✅ 可以对它进行任何类型的重新赋值
thing = false;
thing(); // ✅ 直接作为函数调用
thing.notExist() // ✅ 可以调用任何不存在的方法
正因为any的宽松,请谨慎使用它。
Delayed Initialization & Implicit Any
有一些时候,我们声明变量的确不会马上赋值,如下
const movies = ["Arrival", "The Thing", "Aliens"];
let foundMovie;
那么此时foundMovie
的类型是什么呢? typescript将会如何推断?
我们把鼠标移到foundMovie
便会发现,TS推断它为any
。既然此时为any
,那么此时对foundMovie
做任何操作都是可以的。
const movies = ["Arrival", "The Thing", "Aliens"];
let foundMovie;
for (let movie of movies) {
if (movie === "Amadeus") {
foundMovie = "Amadeus";
}
}
foundMovie(); // ✅ not error
foundMovie = 1; // ✅ not error
foundMovie.abc(); // ✅ not error
此时,我们可以提前为foundMovie
指定类型。
const movies = ["Arrival", "The Thing", "Aliens"];
let foundMovie: string;
for (let movie of movies) {
if (movie === "Amadeus") {
foundMovie = "Amadeus";
}
}
这是一种防止隐式any
的方法。