- Published on
TypeScript - 联合类型
- Authors
- Name
- Deng Hua
目录
Union Types
Union types allow us to give a value a few different possible types. If the eventual value's type is included, Typescript will be happy. We can create a union type by using the single | (pipe character) to separate the types we want to include. It's like saying, "This thing is allowed to be this, this, or this". Typescript will enforce it from there.
联合类型允许我们给一个值多种可能的类型,
let u: string | number | boolean = '123'
u = 1
u = true
当然,对于基本类型,不建议这么使用,应该尽可能的保持严格。
另一个示例
type Point = {
x: number
y: number
}
type Loc = {
lat: number
long: number
}
let coordinates: Point | Loc = { x: 1, y: 34 }
coordinates = { lat: 321.22, long: 233.111}
类型收窄 - Type Narrowing
Narrowing the Type is simply doing a type check before working with a value. If your value is a string you may want to use it differently than if you got a number. Since unions allow multiple types for a value, it's good to check what came through before working with it
function printAge(age: number | string) {
age.toUpperCase() // ❌ 类型“string | number”上不存在属性“toUpperCase”。
}
在这个例子中,TS并不知道此时此刻,参数age
的确定的类型是什么,不要忘了,typescript
只能做静态类型检查,并不能发现运行时的状态。
就像薛定谔的猫
这时候,我们需要做的就是,手动收窄参数的类型。
function printAge(age: number | string) {
if (typeof age === 'string') {
age.toUpperCase();
}
}
如果不做类型收窄,当想调用age上的方法时,可选项只会是number
类型和string
类型的公共方法。
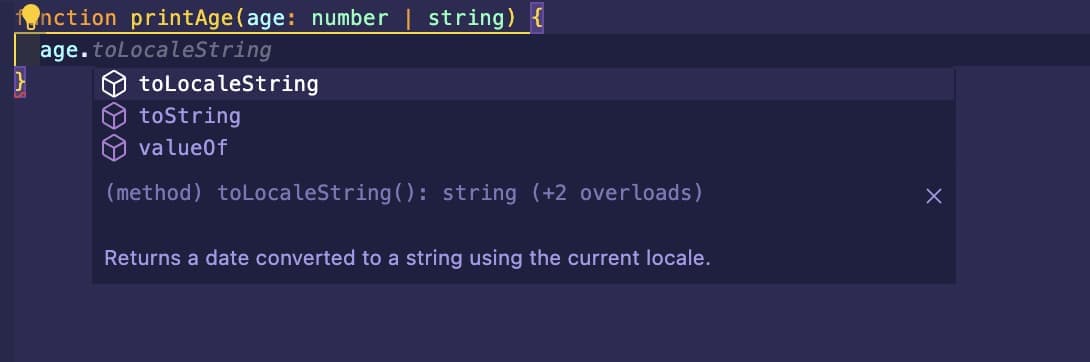
Union Types and Arrays
联合类型也可以与数组类型注释一同使用。
const stuff: (number | string)[] = [1, 'string']
another case
type Sex = 'Male' | 'Female'
type Men = {
name: string
sex: Sex
}
type Women = {
name: string
sex: Sex
}
const person: (Men | Women)[] = [
{ name: 'john', sex: 'Male' },
{ name: 'lisa', sex: 'Female' },
]
字面量类型 - Literal Types
Literal types are not just types - but the values themselves too! On it's own, they may not seem useful, but combine them with unions and you can have very fine-tuned type options for Typescript to enforce.
你可以使用一个字符串字面量作为一个类型:
const hi: 'hi' = 'hi'
与联合类型一并使用,可以约束一些确定范围的值。
const giveAnswer = (answer: 'yes' | 'no') => {
return `The answer is ${answer}`
}
giveAnswer('yes')
giveAnswer('maybe') // ❌ 类型“"maybe"”的参数不能赋给类型“"yes" | "no"”的参数
another case
type DayOfWeek = 'Monday' | 'Tuesday' | 'Wednesday' | 'Thursday' | 'Friday' | 'saturday' | 'Sunday'
const today: DayOfWeek = 'Friday'